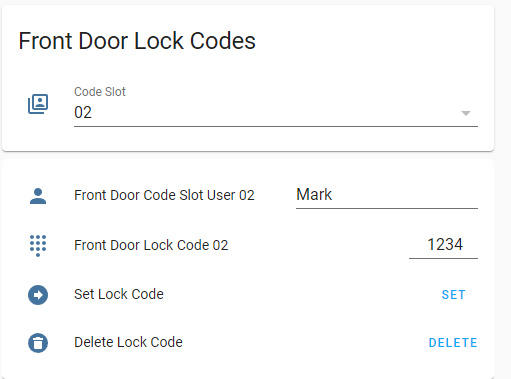
Well with a LOT of help on the templating from @tom_l I was able to modify this script to be a little more user friendly on the front end, as well as include a user name that I can use for presence announcements when some arrives. I thought I would share it, so hopefully someone can take it and make it even better. The original premise is still in place. I created the following helpers for each lock:
->input_select.code_slot (as per the original script w/ 6 code slots for my needs)
->input_text.code_slot_front_user_0x (6 individual Code Slots for each lock to store the individual user names)
->input_number.front_lock_code_0x(6 individual Code Slots for each lock to store the individual user codes)
I ended up putting the following in a package because the script editor kept destroying the code when I put it into the scripts.yaml
script:
set_lock_frontdoor_code:
alias: Set Front Door Lock Code
sequence:
- service: zwave_js.set_lock_usercode
target:
entity_id: lock.lock_front_door
data:
usercode: >
{% if is_state('input_select.code_slot','01') %}
{{ "%04d" % states('input_number.front_lock_code_01')|int }}
{% elif is_state('input_select.code_slot','02') %}
{{ "%04d" % states('input_number.front_lock_code_02')|int }}
{% elif is_state('input_select.code_slot','03') %}
{{ "%04d" % states('input_number.front_lock_code_03')|int }}
{% elif is_state('input_select.code_slot','04') %}
{{ "%04d" % states('input_number.front_lock_code_04')|int }}
{% elif is_state('input_select.code_slot','05') %}
{{ "%04d" % states('input_number.front_lock_code_05')|int }}
{% elif is_state('input_select.code_slot','06') %}
{{ "%04d" % states('input_number.front_lock_code_06')|int }}
{% endif %}
code_slot: '{{ states("input_select.code_slot") | int }}'
mode: single
icon: mdi:arrow-right-bold-circle
delete_lock_frontdoor_code:
alias: Delete Lock Code
sequence:
- service: zwave_js.clear_lock_usercode
target:
entity_id: lock.lock_front_door
data:
code_slot: '{{ states("input_select.code_slot") | int }}'
- service: input_number.set_value
data:
entity_id: >
{% if is_state('input_select.code_slot','01') %}
input_number.front_lock_code_01
{% elif is_state('input_select.code_slot','02') %}
input_number.front_lock_code_02
{% elif is_state('input_select.code_slot','03') %}
input_number.front_lock_code_03
{% elif is_state('input_select.code_slot','04') %}
input_number.front_lock_code_04
{% elif is_state('input_select.code_slot','05') %}
input_number.front_lock_code_05
{% elif is_state('input_select.code_slot','06') %}
input_number.front_lock_code_06
{% endif %}
value: 0
- service: input_text.set_value
data:
entity_id: >
{% if is_state('input_select.code_slot','01') %}
input_text.code_slot_front_user_01
{% elif is_state('input_select.code_slot','02') %}
input_text.code_slot_front_user_02
{% elif is_state('input_select.code_slot','03') %}
input_text.code_slot_front_user_03
{% elif is_state('input_select.code_slot','04') %}
input_text.code_slot_front_user_04
{% elif is_state('input_select.code_slot','05') %}
input_text.code_slot_front_user_05
{% elif is_state('input_select.code_slot','06') %}
input_text.code_slot_front_user_06
{% endif %}
value: ""
mode: single
icon: mdi:delete-circle
The lovelace yaml now needs custom integration: State-Switch
cards:
- type: entities
title: Front Door Lock Codes
entities:
- input_select.code_slot
- type: custom:state-switch
entity: input_select.code_slot
states:
'01':
cards: null
type: entities
entities:
- entity: input_text.code_slot_front_user_01
- entity: input_number.front_lock_code_01
- entity: script.set_lock_frontdoor_code
action_name: Set
type: button
name: Set Lock Code
tap_action:
action: call-service
service: script.set_lock_frontdoor_code
confirmation:
text: Change the lock code for this slot?
- entity: script.delete_lock_frontdoor_code
action_name: Delete
type: button
name: Delete Lock Code
tap_action:
action: call-service
service: script.delete_lock_frontdoor_code
confirmation:
text: Delete the lock code for this slot?
'02':
cards: null
type: entities
entities:
- entity: input_text.code_slot_front_user_02
- entity: input_number.front_lock_code_02
- entity: script.set_lock_frontdoor_code
action_name: Set
type: button
name: Set Lock Code
tap_action:
action: call-service
service: script.set_lock_frontdoor_code
confirmation:
text: Change the lock code for this slot?
- entity: script.delete_lock_frontdoor_code
action_name: Delete
type: button
name: Delete Lock Code
tap_action:
action: call-service
service: script.delete_lock_frontdoor_code
confirmation:
text: Delete the lock code for this slot?
'03':
cards: null
type: entities
entities:
- entity: input_text.code_slot_front_user_03
- entity: input_number.front_lock_code_03
- entity: script.set_lock_frontdoor_code
action_name: Set
type: button
name: Set Lock Code
tap_action:
action: call-service
service: script.set_lock_frontdoor_code
confirmation:
text: Change the lock code for this slot?
- entity: script.delete_lock_frontdoor_code
action_name: Delete
type: button
name: Delete Lock Code
tap_action:
action: call-service
service: script.delete_lock_frontdoor_code
confirmation:
text: Delete the lock code for this slot?
'04':
cards: null
type: entities
entities:
- entity: input_text.code_slot_front_user_04
- entity: input_number.front_lock_code_04
- entity: script.set_lock_frontdoor_code
action_name: Set
type: button
name: Set Lock Code
tap_action:
action: call-service
service: script.set_lock_frontdoor_code
confirmation:
text: Change the lock code for this slot?
- entity: script.delete_lock_frontdoor_code
action_name: Delete
type: button
name: Delete Lock Code
tap_action:
action: call-service
service: script.delete_lock_frontdoor_code
confirmation:
text: Delete the lock code for this slot?
'05':
cards: null
type: entities
entities:
- entity: input_text.code_slot_front_user_05
- entity: input_number.front_lock_code_05
- entity: script.set_lock_frontdoor_code
action_name: Set
type: button
name: Set Lock Code
tap_action:
action: call-service
service: script.set_lock_frontdoor_code
confirmation:
text: Change the lock code for this slot?
- entity: script.delete_lock_frontdoor_code
action_name: Delete
type: button
name: Delete Lock Code
tap_action:
action: call-service
service: script.delete_lock_frontdoor_code
confirmation:
text: Delete the lock code for this slot?
'06':
cards: null
type: entities
entities:
- entity: input_text.code_slot_front_user_06
- entity: input_number.front_lock_code_06
- entity: script.set_lock_frontdoor_code
action_name: Set
type: button
name: Set Lock Code
tap_action:
action: call-service
service: script.set_lock_frontdoor_code
confirmation:
text: Change the lock code for this slot?
- entity: script.delete_lock_frontdoor_code
action_name: Delete
type: button
name: Delete Lock Code
tap_action:
action: call-service
service: script.delete_lock_frontdoor_code
confirmation:
text: Delete the lock code for this slot?
type: vertical-stack
Obviously you would need to create a card and code for each individual lock you want to control, but for me it’s still way less bulky than Keymaster (which I think is a brilliant integration! Just more than I need). This has been tested and confirmed working on my three Yale YRD256 locks. Hopefully it works for you. Good luck!
Thanks to @dposluns for sharing the original code and inspiration behind my hack! 