HI All,
what I am trying to find out, coming from legacy HA, is how this new animated card for Lovelace can show the state of the weather component in the header.
I thought this line:
<span class="icon bigger" style="background: none, url(/local/weather/animated/${weatherIcons[currentCondition]}.svg) no-repeat; background-size: contain;"> ${currentCondition}</span>
should take care of that, but it doesn’t. Not sure why the ${currentCondition is there, should it popup hovering the icon (it doesn’t in my setup) ?
Anyways, as @arsaboo shows in his initial post of this thread, this is what I am referring to:
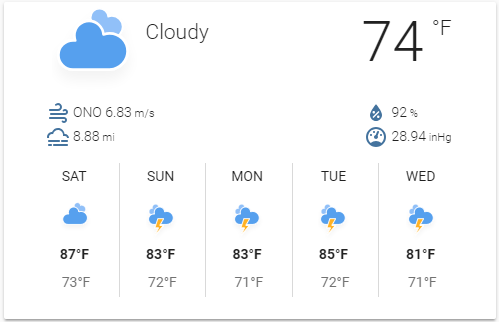
mine is now showing like this, and Id love to have the state back in…
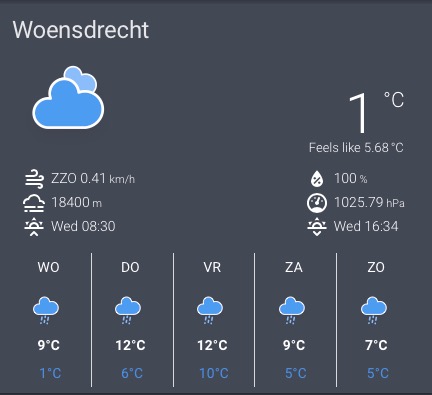
btw I am using weather Buienradar, and was severely helped by @jusdwy in this thread about the dark sky card Custom Dark Sky Animated Weather Card
Please have a look how to show the entity.state, and for reference sake below is my js file.
class WeatherCard extends HTMLElement {
set hass(hass) {
if (!this.content) {
const card = document.createElement('ha-card');
const link = document.createElement('link');
card.header = hass.states[this.config.entity_weather].attributes.friendly_name;
link.type = 'text/css';
link.rel = 'stylesheet';
link.href = '/local/lovelace/weather-card.css';
card.appendChild(link);
this.content = document.createElement('div');
this.content.className = 'card';
card.appendChild(this.content);
this.appendChild(card);
}
const getUnit = function (measure) {
const lengthUnit = hass.config.unit_system.length;
switch (measure) {
case 'air_pressure':
return lengthUnit === 'km' ? 'hPa' : 'inHg';
case 'length':
return lengthUnit;
case 'visibility':
return lengthUnit === 'km' ? 'm' : 'in';
case 'precipitation':
return lengthUnit === 'km' ? 'mm' : 'in';
default:
return hass.config.unit_system[measure] || '';
}
};
const transformDayNight = {
"below_horizon": "night",
"above_horizon": "day",
}
const sunLocation = transformDayNight[hass.states[this.config.entity_sun].state];
const weatherIcons = {
'clear-night': `${sunLocation}`,
'cloudy': 'cloudy',
'fog': 'cloudy',
'hail': 'rainy-7',
'lightning': 'thunder',
'lightning-rainy': 'thunder',
'partlycloudy': `cloudy-${sunLocation}-3`,
'pouring': 'rainy-6',
'rainy': 'rainy-5',
'snowy': 'snowy-6',
'snowy-rainy': 'rainy-7',
'sunny': `${sunLocation}`,
'windy': 'cloudy',
'windy-variant': `cloudy-${sunLocation}-3`,
'exceptional': '!!',
}
const windDirections = ['N','NNO','NO','ONO',
'O','OZO','ZO','ZZO',
'Z','ZZW','ZW','WZW',
'W','WNW','NW','NNW',
'N'];
const entity = hass.states[this.config.entity_weather];
const currentCondition = entity.state;
const humidity = entity.attributes.humidity;
const pressure = entity.attributes.pressure;
const temperature = entity.attributes.temperature;
const visibility = entity.attributes.visibility;
const windBearing = windDirections[(parseInt((entity.attributes.wind_bearing + 11.25) / 22.5))];
const windSpeed = entity.attributes.wind_speed;
const forecast = entity.attributes.forecast.slice(0, 5);
const apparent_temperature = hass.states[this.config.entity_apparent_temperature].state;
// const sunrise = hass.states[this.config.entity_sun].attributes.next_rising;
// const sunset = hass.states[this.config.entity_sun].attributes.next_setting;
var sunSetOrRiseA = new Date(hass.states[this.config.entity_sun].attributes.next_setting);
var sunSetOrRiseIconA = "mdi:weather-sunset-down";
var sunSetOrRiseB = new Date(hass.states[this.config.entity_sun].attributes.next_rising);
var sunSetOrRiseIconB = "mdi:weather-sunset-up";
// A == sunset B == sunrise
if ( hass.states[this.config.entity_sun].state == "above_horizon" ) {
// sun has risen, but hasn't set
// sunset is today (no date displayed). sunrise is tomorrow (display date)
// next is sunset == A
sunSetOrRiseA = sunSetOrRiseA.toLocaleTimeString();
var ssrI = sunSetOrRiseA.lastIndexOf(":");
sunSetOrRiseA = sunSetOrRiseA.substr(0,ssrI) + sunSetOrRiseA.substr(ssrI+4);
sunSetOrRiseB = sunSetOrRiseB.toDateString().substr(0,3) + " " + sunSetOrRiseB.toLocaleTimeString();
ssrI = sunSetOrRiseB.lastIndexOf(":");
sunSetOrRiseB = sunSetOrRiseB.substr(0,ssrI) + sunSetOrRiseB.substr(ssrI+4);
} else {
// next is sunrise == B
var ss = sunSetOrRiseA;
if ( new Date().getDate() != sunSetOrRiseB.getDate() ) {
// sun hasn't risen, and it's not same day
// so display dates for both
sunSetOrRiseA = sunSetOrRiseB.toDateString().substr(0,3) + " " + sunSetOrRiseB.toLocaleTimeString();
sunSetOrRiseB = ss.toDateString().substr(0,3) + " " + ss.toLocaleTimeString();
} else {
// sun hasn't risen, but it's the same day
// since rise and set are today, no dates displayed
sunSetOrRiseA = sunSetOrRiseB.toLocaleTimeString();
sunSetOrRiseB = ss.toLocaleTimeString();
}
var ssrI = sunSetOrRiseA.lastIndexOf(":");
sunSetOrRiseA = sunSetOrRiseA.substr(0,ssrI) + sunSetOrRiseA.substr(ssrI+4);
ssrI = sunSetOrRiseB.lastIndexOf(":");
sunSetOrRiseB = sunSetOrRiseB.substr(0,ssrI) + sunSetOrRiseB.substr(ssrI+4);
sunSetOrRiseIconA = "mdi:weather-sunset-up";
sunSetOrRiseIconB = "mdi:weather-sunset-down";
}
this.content.innerHTML = `
<span class="icon bigger" style="background: none, url(/local/weather/animated/${weatherIcons[currentCondition]}.svg) no-repeat; background-size: contain;"> ${currentCondition}</span>
<ul style="list-style-type:none">
<li><span class="temp">${temperature}</span><span class="tempc"> ${getUnit('temperature')}</span></li>
<li><span class="tempa">Feels like ${apparent_temperature}</span><span class="unitc"> ${getUnit('temperature')}</span></li>
</ul>
<span>
<ul class="variations right">
<li><span class="ha-icon"><ha-icon icon="mdi:water-percent"></ha-icon></span>${humidity}<span class="unit"> %</span></li>
<li><span class="ha-icon"><ha-icon icon="mdi:gauge"></ha-icon></span>${pressure}<span class="unit"> ${getUnit('air_pressure')}</span></li>
<li><span class="ha-icon"><ha-icon icon=${sunSetOrRiseIconB}></ha-icon></span>${sunSetOrRiseB}</li>
</ul>
<ul class="variations">
<li><span class="ha-icon"><ha-icon icon="mdi:weather-windy"></ha-icon></span>${windBearing} ${windSpeed}<span class="unit"> ${getUnit('length')}/h</span></li>
<li><span class="ha-icon"><ha-icon icon="mdi:weather-fog"></ha-icon></span>${visibility}<span class="unit"> ${getUnit('visibility')}</span></li>
<li><span class="ha-icon"><ha-icon icon=${sunSetOrRiseIconA}></ha-icon></span>${sunSetOrRiseA}</li>
</ul>
</span>
<div class="forecast clear">
${forecast.map(daily => `
<div class="day">
<span class="dayname">${(new Date(daily.datetime)).toLocaleDateString((navigator.language) ? navigator.language : navigator.userLanguage, {weekday: 'short'}).split(' ')[0]}</span>
<br><i class="icon" style="background: none, url(/local/weather/animated/forecast/${weatherIcons[daily.condition]}.svg) no-repeat; background-size: contain;"></i>
<br><span class="highTemp">${daily.temperature}${getUnit('temperature')}</span>
<br><span class="lowTemp">${daily.templow}${getUnit('temperature')}</span>
</div>`).join('')}
</div>`;
}
setConfig(config) {
if (!config.entity_weather || !config.entity_sun) {
throw new Error('Please define entities');
}
this.config = config;
}
// @TODO: This requires more intelligent logic
getCardSize() {
return 3;
}
}
customElements.define('weather-card', WeatherCard);
// <li><span class="ha-icon"><ha-icon icon="mdi:weather-sunset-up"></ha-icon></span>${sunrise}<span class="unitc"> ${getUnit('sunrise')}</span></li>
// <li><span class="ha-icon"><ha-icon icon="mdi:weather-sunset-down"></ha-icon></span>${sunset}<span class="unitc"> ${getUnit('sunset')}</span></li>
—edit----
been hacking the css and main .js file a bit further and now have this:
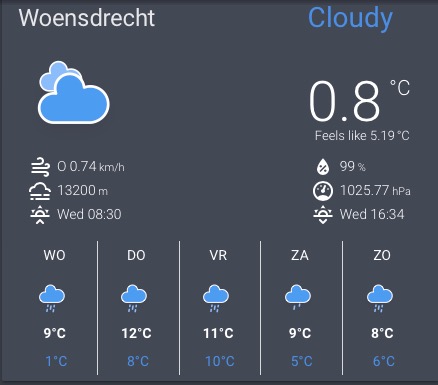
well enough for today…