Well, keeping in mind that I am an AD newbie myself, hereās what I did:
First I set up Vassilisā cool custom component per this thread. This creates a media player component that you use to change channels by using the āmedia_player.select_sourceā service. For example, in the case of my Dish Network Joey, I have channels 0-9 set up like this:
chan_01 = JgBIAA3EDF0LXQxdDDYLXQxdC10LXg1cDFwMXQtdC14LXgtdDVwLxQxeDFwMXQs2C10MXQxdC10NXAtdC14MXQxcDF0LXQteDAANBQ==
chan_02 = JgBIAAzFDF0NWwteCzYLXg00DF0LXQxdC10MXQxdDFwOWwtdDF0Mww5dC10MXQs2C14LNgxdDVsMXQtdDF0MXQtdDF0LXQxdDAANBQ==
chan_03 = JgBIAAvFDV0MXAtdDDYLNgxdC10MXQxdC10LXgtdDF0LXQxdC14LxgxdC10LXQw2CzYMXQxdDVsMXQtdDF0MXQtdDF0MXAteDQANBQ==
chan_04 = JgBIAA3GDlsLXQs3DFwMXA5bDF0NWwxdC10OWw1cDFwMXQtdDlsMxQxdC10OMg5bDlwLXQxdC10MXQxdDFwMXQtdDlsNXAtdDAANBQ==
chan_05 = JgBIAAzGC10MXQs2C14LXQw1DF0NXAtdDF0LXQxdDF0MXAxdC10NxQtdDVwLNgteC10NNA1cDVwLXQ1cC10MXQxdDFwMXQxdDAANBQ==
chan_06 = JgBIAA7FDF0MXAw2DFwLNwtdDF0MWg5dC14LXQxdC14LXQteC10NxAxdC10MNgtdDDYLXQxdDF0NWwteC10NXAxdC10NXAtdDAANBQ==
chan_07 = JgBIAAvFDF0MXQs2DDYMXAxdC10NXAxdC10LXgtdDF0MXQtdC14LxgteDFwLNgw2C10MXQxdC10MXQtdC14MXQxcC14LXQteCwANBQ==
chan_08 = JgBIAAvGDF0LXQw2DTQNXAs2C10MXQxdC10MXQtdDF0NXAtdDF0LxgxdC10MNgw1C14LNgtdDF0NXAxcDVwLXQxdDF0NWwxdCwANBQ==
chan_09 = JgBIAAzFDF0MXQ00DDYLNgteDFwLXQ1cDVwLXgtdC10MXQxdDFwMxQxdDF0LNgw2DDUMXQtdC14LXQxdC14NWwtdDF0MXQtdDAANBQ==
chan_00 = JgBIAA3EDF0MNQxdC10MXQw1DVwMXQtdC14LXQ5bC14MXAtdDF0NxAxdCzYMXQxcDF0MNQxdDF0LXQteC10MXQ1cDFwLXgtdDQANBQ==
And my media player is set up like:
- platform: broadlink
name: Office TV & Dish
host: 192.168.0.132
mac: 'XX:XX:XX:XX:XX:XX'
ircodes_ini: '/devices/broadlink/broadlink_media_codes/office.ini'
Next I set up an IFTTT applet that uses webhooks and the HASS API like this:
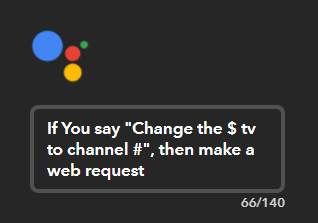
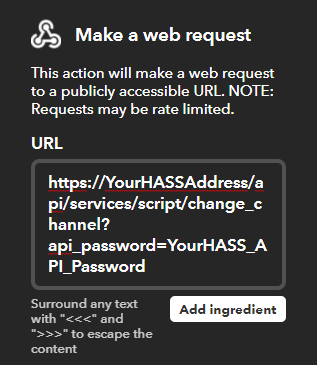
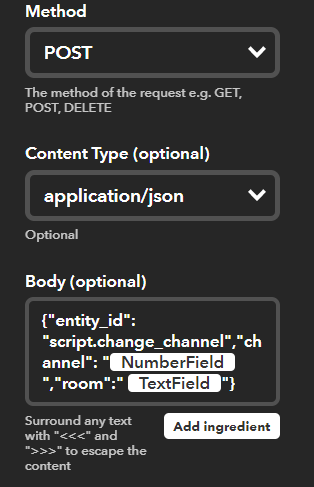
The IFTTT applet calls a HASS script (change_channel.yaml) that consists of:
alias: "Change the Dish channel"
sequence:
- service: python_script.set_channel
data_template:
channel: "{{channel}}"
room: "{{room}}"
This, in turn, calls the HASS python script (set_channel.py) below:
channel = data.get('channel')
#for some reason IFTT passes the word "the" when sending "Change the $ tv to channel #" so strip out the word "the"
room = data.get('room').replace('the ', '')
#replace any spaces in the room name with underscores
room = room.replace(" ","_")
hass.bus.fire("change_channel", {"channel": channel, "room": room})
Once the change channel event fires, itās picked up by the appdaemon script (set_channel.py) below:
import appdaemon.plugins.hass.hassapi as hass
#
# Change Channel on Dish Receiver
# To call it, fire a change_channel event with a "channel" and a "room" param
#
class SetChannel(hass.Hass):
def initialize(self):
self.log("SetChannel loaded")
self.listen_event(self.do_change, 'change_channel')
def do_change(self, event_name, data, kwargs):
delay = 1
for c in str(data["channel"]):
if delay == 0:
self.do_channel({"channel":c, "room":data["room"]})
else:
self.run_in(self.do_channel, delay, channel = c, room = data["room"])
delay += 1
def do_channel(self, kwargs):
self.call_service("media_player/select_source", source = "chan_0"+kwargs["channel"], entity_id = "media_player."+kwargs["room"]+"_tv__dish")
which then calls the media_player.select_source service sending the concatenated source and entity_id.
Itās a real Rube Goldberg machine that Iām sure can be improved upon a lot but it works well.
Hopefully that covers it all. Good luck!