Hi all,
I’ve been struggling getting the deluge integration to work, so I wrote an appdaemon script which monitors the download and upload speeds as well as torrent status (# of active, seeding, paused, queued, etc torrents). Idk if anyone has gotten the integration to work, but since it offers only speed monitoring, this was not sufficient in my case. Anyhow below is the code I wrote and can be easily extended to monitor additional variables of choice or even to actively modify torrent conditions (set speed limits, pause them etc.).
import hassapi as hass
import requests
from datetime import datetime
class Deluge(hass.Hass):
prefix = 'deluge_'
url = 'http://host-ip:8112/json'
headers = {
'Content-Type': 'application/json',
'Accept': 'application/json'
}
cookies = None
handle = None
def login(self):
body = {
"method": "auth.login",
"params": ["deluge"],
"id": 2
}
response = requests.post(
self.url,
json=body,
headers=self.headers,
)
json_response = response.json()
if(json_response['result']):
return response.cookies
else:
self.log('Deluge: could not log in!')
return json_response['result']
def initialize(self):
self.log("Starting Deluge")
tm = datetime.now()
if self.handle != None:
self.handle = self.run_minutely(self.runMain, tm)
else:
self.cancel_timer(self.handle)
self.handle = self.run_minutely(self.runMain, tm)
def runMain(self, kwargs):
if(self.isConnected()):
res = self.updateWebUI()
params = self.getParams(res)
self.updateHAStates(params)
self.log('Updated HA...')
else:
self.log('Connecting...')
cookie = self.login()
if(cookie):
self.cookies = cookie
def isConnected(self):
body_con = {
"method": "web.connected",
"params": [],
"id": 2
}
response = requests.post(
self.url,
cookies=self.cookies,
json=body_con,
headers=self.headers
)
json_response = response.json()
return json_response['result']
def updateWebUI(self):
body_ui = {
"method": "web.update_ui",
"params": [{},None],
"id": 2
}
response = requests.post(
self.url,
cookies=self.cookies,
json=body_ui,
headers=self.headers
)
json_response = response.json()
return json_response['result']
def getParams(self,res):
dl = float(res['stats']['download_rate'])/1024
ul = float(res['stats']['upload_rate'])/1024
torrents_all = res['filters']['state'][0][1]
torrents_active = res['filters']['state'][1][1]
torrents_downloading = res['filters']['state'][4][1]
torrents_seeding = res['filters']['state'][5][1]
torrents_paused = res['filters']['state'][6][1]
torrents_queued = res['filters']['state'][8][1]
return {'dl': dl,
'ul': ul,
'torrents_all':torrents_all,
'torrents_active':torrents_active,
'torrents_downloading':torrents_downloading,
'torrents_seeding':torrents_seeding,
'torrents_paused':torrents_paused,
'torrents_queued':torrents_queued
}
def updateHAStates(self,params):
for ix,e in params.items():
self.set_state("input_number."+self.prefix+ix, state=e)
The only things that you need to change in the script is the host-ip of the device running deluge and the password to the web-ui. Also you need to have the web-ui daemon running. The script runs minutely and upon completion will add a number of variables in the form of “input_number.deluge_variables” for download and upload speed and torrent statuses. Those can can be integrated within a visualization as such:
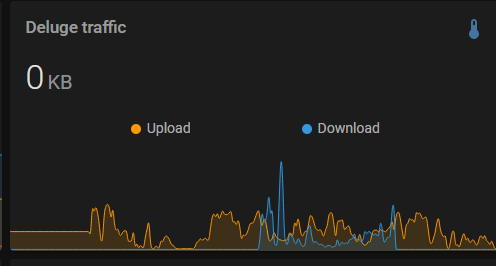
edit: as I was using the code, I noticed some inefficiencies and updated the code here with the latest revision.