maybe we can overcome this too using the Python.
I need an attribute ‘text’ with the outcome of the sensor as value. So where the regular jinja template sensor is:
map_totaal_actueel_sensors:
friendly_name: Overzicht sensors actueel
unit_of_measurement: 'Watt'
value_template: >
{%-for state in states.sensor
if state.entity_id.endswith('actueel') and state.state|float > 0 %}
{{state.name[:-8]}}: {{state.state}}
{%-endfor%}
{{ '-'.ljust(30, '-') }} {{ '-'.ljust(30, '-') }}
'Count sensors :' {{ state_attr('sensor.total_sensors', 'count') }} 'totaal: ' {{ states.sensor|selectattr( 'entity_id','in',state_attr('group.iungo_actueel_verbruik','entity_id'))
|map(attribute='state')|map('int')|sum }}
id need that to be calculated in Python, and be the value for text.
I can then create the sensor in Python as follows:
hass.states.set(
‘sensor.map_totaal_actueel_sensors’, ‘’, {
‘custom_ui_state_card’: ‘state-card-value_only’,
‘text’: outcome-of-template-here
})
I think an attribute ‘text’ cant be set in a regular sensor? If so that would be even easier to try.
Would you please have another go for me? Seems we have almost all already, the count, the total power usage, and the states.
Only thing left in this nicely printed overview is the formatting.
##########################################################################################
# map_total_sensors.py
# reading all sensors.*_actueel using power (>0), listing and counting them, and
# calculate summed power consumption
# by @Mariusthvdb and big hand Phil, @pnbruckner
##########################################################################################
sensor_list = []
total_power = 0
count = 0
for entity_id in hass.states.entity_ids('sensor'):
if entity_id.endswith('actueel'):
state = hass.states.get(entity_id)
logger.debug('entity_id = {}, state = {}'.format(entity_id, state.state))
sensor = '{} : {}'.format(entity_id, state.state)
try:
power = float(state.state)
except:
continue
if power > 0:
total_power = round((total_power + power),2)
count = count + 1
sensor_list.append(sensor)
summary = '*============== Sensors ==============\n' \
'----- Sensor ------------ Power -----\n' \
'${}\n' \
'*=============== Sumary ==============\n' \
'$ Sensors {} : Total Power {}\n' \
'*=====================================\n' \
.format(sensor_list,
count,
total_power)
hass.states.set('sensor.map_total_sensors', '', {
'custom_ui_state_card': 'state-card-value_only',
'text': summary
})
##########################################################################################
# map_total_sensors.py
##########################################################################################
showing as:
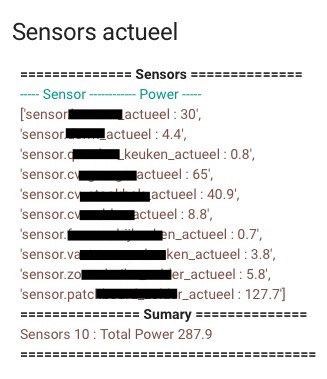
quite the result already, and updating immediately, both per sensor, as the total.
changed is somewhat to do away with the opening and closing list brackets, and the apostrophes around each sensor. As often the case, one needs intermediate variables, and a bit of playing with join, append and format…
This way each sensor gets and stays on its own line too, which is very nice when changing the browser window. (before it filled out, since the sensor section was seen as 1 entity.)
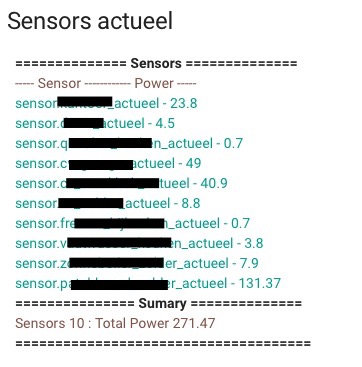
changed code:
sensor_list = []
total_power = 0
count = 0
for entity_id in hass.states.entity_ids('sensor'):
if entity_id.endswith('actueel'):
state = hass.states.get(entity_id)
logger.debug('entity_id = {}, state = {}'.format(entity_id, state.state))
#sensor_id = '{}'.format(entity_id)
#sensor_power = '{}'.format(state.state)
#sensor = '{} - {}\n'.format(sensor, senor_power)
sensor = '{} - {}\n'.format(entity_id[7:-8], state.state)
try:
power = float(state.state)
except:
continue
if power > 0:
total_power = round((total_power + power),2)
count = count + 1
sensor_list.append(sensor)
sensor_map = '\n'.join(sensor_list)
summary = '*============== Sensors ==============\n' \
'$----- Sensor ------------ Power -----\n' \
'{}\n' \
'*=============== Sumary ==============\n' \
'$ Sensors {} : Total Power {}\n' \
'*=====================================\n' \
.format(sensor_map,
count,
total_power)
Of course ill need to reformat the rest of the map too, using some of the color options in the custom card, and better justification, as in the original jinja template.