I have a goal of displaying external data so that I don’t need to include my weather data into homeassistant (and can have a long term graph) and today we succeeded in doing so with a custom web server. But I do get interesting results displayed.
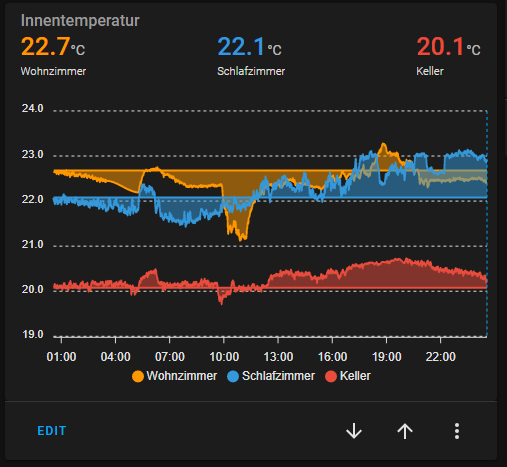
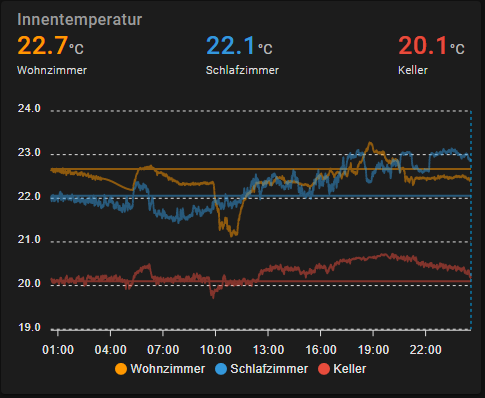
Using (almost) the same data via mqtt sensor works like it should
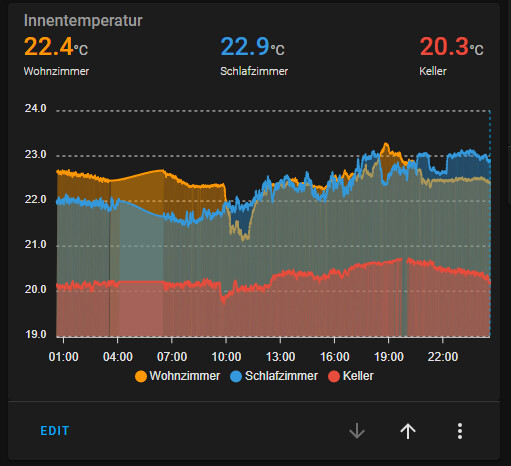
This is the complete code of the data generator card:
type: custom:apexcharts-card
graph_span: 1d
hours_12: false
header:
show: true
title: Innentemperatur
show_states: true
colorize_states: true
standard_format: false
now:
show: true
all_series_config:
type: area
stroke_width: 2
opacity: 0.5
unit: °C
show:
legend_value: false
series:
- entity: sensor.time
name: Wohnzimmer
data_generator: |
function makeRequest(method, url) {
return new Promise(function (resolve, reject) {
let xhr = new XMLHttpRequest();
xhr.open(method, url);
xhr.onload = function () {
if (this.status >= 200 && this.status < 300) {
resolve(xhr.response);
} else {
reject({
status: this.status,
statusText: xhr.statusText
});
}
};
xhr.onerror = function () {
reject({
status: this.status,
statusText: xhr.statusText
});
};
xhr.send();
});
}
const request = async () => {
var http = new XMLHttpRequest();
var response = await makeRequest('GET', 'https://kuroi1992.dyndns.pro/test?type=temperature&location=inside&name=wohnzimmer&time=24h');
console.debug(response);
var json = JSON.parse(response);
console.debug(json);
return json.map(x => {
return [x['time'], x['value']];
});
}
return request();
- entity: sensor.time
name: Schlafzimmer
data_generator: |
function makeRequest(method, url) {
return new Promise(function (resolve, reject) {
let xhr = new XMLHttpRequest();
xhr.open(method, url);
xhr.onload = function () {
if (this.status >= 200 && this.status < 300) {
resolve(xhr.response);
} else {
reject({
status: this.status,
statusText: xhr.statusText
});
}
};
xhr.onerror = function () {
reject({
status: this.status,
statusText: xhr.statusText
});
};
xhr.send();
});
}
const request = async () => {
var http = new XMLHttpRequest();
var response = await makeRequest('GET', 'https://kuroi1992.dyndns.pro/test?type=temperature&location=inside&name=schlafzimmer&time=24h');
console.debug(response);
var json = JSON.parse(response);
console.debug(json);
return json.map(x => {
return [x['time'], x['value']];
});
}
return request();
- entity: sensor.time
name: Keller
data_generator: |
function makeRequest(method, url) {
return new Promise(function (resolve, reject) {
let xhr = new XMLHttpRequest();
xhr.open(method, url);
xhr.onload = function () {
if (this.status >= 200 && this.status < 300) {
resolve(xhr.response);
} else {
reject({
status: this.status,
statusText: xhr.statusText
});
}
};
xhr.onerror = function () {
reject({
status: this.status,
statusText: xhr.statusText
});
};
xhr.send();
});
}
const request = async () => {
var http = new XMLHttpRequest();
var response = await makeRequest('GET', 'https://kuroi1992.dyndns.pro/test?type=temperature&location=inside&name=keller&time=24h');
console.debug(response);
var json = JSON.parse(response);
console.debug(json);
return json.map(x => {
return [x['time'], x['value']];
});
}
return request();
apex_config:
markers:
hover:
size: 5
Before anyone suggests it, yes, the same problem happens even if I use the sensors corresponding to the temperature sensor, I just replaced them with sensor.time because in the long run I want to run completely via data generator and I needed to test if it works with any random sensor.
I have another graph using the same code and web backend with similar temperatures and on thet one it displays correctly so far.
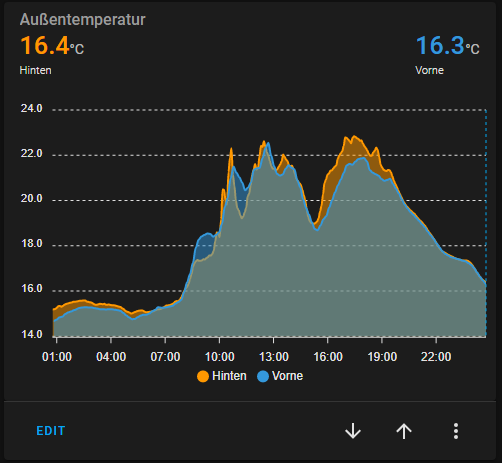
The webserver used for testing is not online most of the time, so if anyone tries to test the example ot, it most likely won’t work.
Anyone got any idea how to force the curves to display correctly? I did try to manually set a minimum for the yaxis, but that only moved the yaxis, but still didn’t render the curves down to the axis.