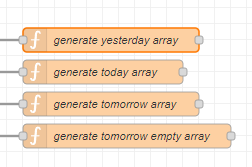
In the same order:
var ypriceArray = [];
for (let i = 0; i < 24; i++) {
ypriceArray[i] = Math.round(100 * (0.21 + 2.79372 +4.56+0.1*1.24*(msg.payload.Publication_MarketDocument.TimeSeries[0].Period[0].Point[i]["price.amount"][0])))/100;
}
flow.set("ypArray", ypriceArray);
msg.payload = { "yday_ahead_price": ypriceArray };
return msg;
var priceArray = [];
for (let i = 0; i < 24; i++) {
priceArray[i] = Math.round(100 * (0.21 + 2.79372 + 4.56 + 0.1 * 1.24 *(msg.payload.Publication_MarketDocument.TimeSeries[0].Period[0].Point[i]["price.amount"][0]))) / 100;
}
flow.set("pArray", priceArray);
msg.payload = { "day_ahead_price": priceArray };
return msg;
var tpriceArray = [];
for (let i = 0; i < 24; i++) {
tpriceArray[i] = Math.round(100 * (0.21 + 2.79372 + 4.56 + 0.1 * 1.24 *(msg.payload.Publication_MarketDocument.TimeSeries[0].Period[0].Point[i]["price.amount"][0]))) / 100;
}
flow.set("tpArray", tpriceArray);
msg.payload = { "tday_ahead_price": tpriceArray };
return msg;
var tpriceArray = [];
for (let i = 0; i < 24; i++) {
tpriceArray[i] = 0
}
flow.set("tpArray", tpriceArray);
msg.payload = { "tday_ahead_price": tpriceArray };
return msg;
Pls. note that here: Math.round(100 * (0.21 + 2.79372 + 4.56 + 0.1 * 1.24 *(msg.payload.Publication_MarketDocument.TimeSeries[0].Period[0].Point[i]["price.amount"][0]))) / 100;
I have various other kWh-based costs like electricity company marginal (0.21), tax (2.79372), transfer company fee (4.56) + then some scaling and the VAT (1.24).
This means my arrays have all the kWh-based costs, you need to do your own stuff here.
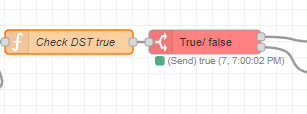
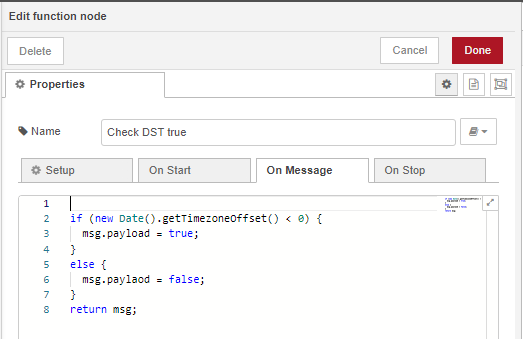
if (new Date().getTimezoneOffset() < 0) {
msg.payload = true;
}
else {
msg.paylaod = false;
}
return msg;
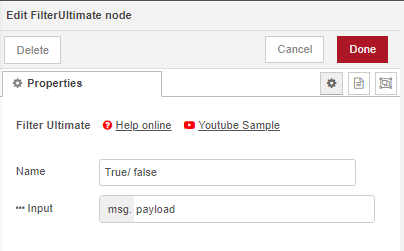
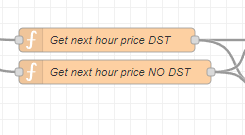
In the same order:
var priceArray = [];
var ypriceArray = [];
var tpriceArray = [];
var c_day_ahead_price;
var max_today;
var min_today;
priceArray = flow.get("pArray");
ypriceArray = flow.get("ypArray");
tpriceArray = flow.get("tpArray");
const today = new Date();
let todayHour = today.getHours();
let todayDate = ("0" + today.getDate()).slice(-2)
let todayMonth = ("0" + (today.getMonth() + 1)).slice(-2);
let todayYear = today.getFullYear();
const tomorrow = new Date()
tomorrow.setDate(tomorrow.getDate() + 1);
let tomorrowDate = ("0" + tomorrow.getDate()).slice(-2)
let tomorrowMonth = ("0" + (tomorrow.getMonth() + 1)).slice(-2);
let tomorrowYear = tomorrow.getFullYear();
let tomorrowString = tomorrowYear + tomorrowMonth + tomorrowDate;
max_today = (Math.max(...priceArray, ypriceArray[23]));
min_today = (Math.min(...priceArray, ypriceArray[23]));
if (todayHour < 1) {
c_day_ahead_price = ypriceArray[23];
} else {
c_day_ahead_price = priceArray[todayHour - 1];
}
msg.payload = {
"day_ahead_price": c_day_ahead_price,
"max_today": max_today,
"min_today": min_today,
"records": [
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T00:00:00",
"Price": ypriceArray[23]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T01:00:00",
"Price": priceArray[0]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T02:00:00",
"Price": priceArray[1]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T03:00:00",
"Price": priceArray[2]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T04:00:00",
"Price": priceArray[3]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T05:00:00",
"Price": priceArray[4]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T06:00:00",
"Price": priceArray[5]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T07:00:00",
"Price": priceArray[6]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T08:00:00",
"Price": priceArray[7]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T09:00:00",
"Price": priceArray[8]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T10:00:00",
"Price": priceArray[9]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T11:00:00",
"Price": priceArray[10]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T12:00:00",
"Price": priceArray[11]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T13:00:00",
"Price": priceArray[12]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T14:00:00",
"Price": priceArray[13]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T15:00:00",
"Price": priceArray[14]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T16:00:00",
"Price": priceArray[15]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T17:00:00",
"Price": priceArray[16]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T18:00:00",
"Price": priceArray[17]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T19:00:00",
"Price": priceArray[18]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T20:00:00",
"Price": priceArray[19]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T21:00:00",
"Price": priceArray[20]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T22:00:00",
"Price": priceArray[21]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T23:00:00",
"Price": priceArray[22]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T00:00:00",
"Price": priceArray[23]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T01:00:00",
"Price": tpriceArray[0]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T02:00:00",
"Price": tpriceArray[1]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T03:00:00",
"Price": tpriceArray[2]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T04:00:00",
"Price": tpriceArray[3]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T05:00:00",
"Price": tpriceArray[4]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T06:00:00",
"Price": tpriceArray[5]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T07:00:00",
"Price": tpriceArray[6]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T08:00:00",
"Price": tpriceArray[7]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T09:00:00",
"Price": tpriceArray[8]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T10:00:00",
"Price": tpriceArray[9]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T11:00:00",
"Price": tpriceArray[10]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T12:00:00",
"Price": tpriceArray[11]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T13:00:00",
"Price": tpriceArray[12]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T14:00:00",
"Price": tpriceArray[13]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T15:00:00",
"Price": tpriceArray[14]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T16:00:00",
"Price": tpriceArray[15]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T17:00:00",
"Price": tpriceArray[16]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T18:00:00",
"Price": tpriceArray[17]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T19:00:00",
"Price": tpriceArray[18]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T20:00:00",
"Price": tpriceArray[19]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T21:00:00",
"Price": tpriceArray[20]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T22:00:00",
"Price": tpriceArray[21]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T23:00:00",
"Price": tpriceArray[22]
}
]
};
return msg;
var priceArray = [];
var tpriceArray = [];
var max_today;
var min_today;
priceArray = flow.get("pArray");
const today = new Date();
let todayHour = today.getHours();
let todayDate = ("0" + today.getDate()).slice(-2)
let todayMonth = ("0" + (today.getMonth() + 1)).slice(-2);
let todayYear = today.getFullYear();
const tomorrow = new Date()
tomorrow.setDate(tomorrow.getDate() + 1);
let tomorrowDate = ("0" + tomorrow.getDate()).slice(-2)
let tomorrowMonth = ("0" + (tomorrow.getMonth() + 1)).slice(-2);
let tomorrowYear = tomorrow.getFullYear();
let tomorrowString = tomorrowYear + tomorrowMonth + tomorrowDate;
max_today = (Math.max(...priceArray));
min_today = (Math.min(...priceArray));
msg.payload = {
"day_ahead_price": priceArray[todayHour],
"max_today": max_today,
"min_today": min_today,
"records": [
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T00:00:00",
"Price": priceArray[0]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T01:00:00",
"Price": priceArray[1]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T02:00:00",
"Price": priceArray[2]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T03:00:00",
"Price": priceArray[3]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T04:00:00",
"Price": priceArray[4]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T05:00:00",
"Price": priceArray[5]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T06:00:00",
"Price": priceArray[6]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T07:00:00",
"Price": priceArray[7]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T08:00:00",
"Price": priceArray[8]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T09:00:00",
"Price": priceArray[9]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T10:00:00",
"Price": priceArray[10]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T11:00:00",
"Price": priceArray[11]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T12:00:00",
"Price": priceArray[12]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T13:00:00",
"Price": priceArray[13]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T14:00:00",
"Price": priceArray[14]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T15:00:00",
"Price": priceArray[15]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T16:00:00",
"Price": priceArray[16]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T17:00:00",
"Price": priceArray[17]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T18:00:00",
"Price": priceArray[18]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T19:00:00",
"Price": priceArray[19]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T20:00:00",
"Price": priceArray[20]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T21:00:00",
"Price": priceArray[21]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T22:00:00",
"Price": priceArray[22]
},
{
"Time": todayYear + "-" + todayMonth + "-" + todayDate + "T23:00:00",
"Price": priceArray[23]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T00:00:00",
"Price": tpriceArray[0]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T01:00:00",
"Price": tpriceArray[1]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T02:00:00",
"Price": tpriceArray[2]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T03:00:00",
"Price": tpriceArray[3]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T04:00:00",
"Price": tpriceArray[4]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T05:00:00",
"Price": tpriceArray[5]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T06:00:00",
"Price": tpriceArray[6]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T07:00:00",
"Price": tpriceArray[7]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T08:00:00",
"Price": tpriceArray[8]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T09:00:00",
"Price": tpriceArray[9]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T10:00:00",
"Price": tpriceArray[10]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T11:00:00",
"Price": tpriceArray[11]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T12:00:00",
"Price": tpriceArray[12]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T13:00:00",
"Price": tpriceArray[13]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T14:00:00",
"Price": tpriceArray[14]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T15:00:00",
"Price": tpriceArray[15]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T16:00:00",
"Price": tpriceArray[16]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T17:00:00",
"Price": tpriceArray[17]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T18:00:00",
"Price": tpriceArray[18]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T19:00:00",
"Price": tpriceArray[19]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T20:00:00",
"Price": tpriceArray[20]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T21:00:00",
"Price": tpriceArray[21]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T22:00:00",
"Price": tpriceArray[22]
},
{
"Time": tomorrowYear + "-" + tomorrowMonth + "-" + tomorrowDate + "T23:00:00",
"Price": tpriceArray[23]
}
]
};
return msg;
Try with these.